Fonts!
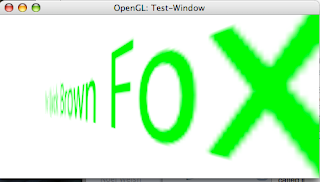
Fonts under Mac OS X are kind of confusing to me. Tasks that seem like they should be easy (such as finding a font, or rendering text using a particular font) usually require you to navigate through several layers of code. I'm sure this is much easier if you are working with X-Code, but as I am tinkering with OpenMCL using VIM and a console for testing, it's often hard to find the specific classes and methods necessary to do what I want.
For example, attempting to determine the width of a particular string (in pixels) for a given font, etc., requires a fairly hefty chunk of Objc-Bridge code:
;;
;; Some AD3D stuff. A Font-Spec is a list: (list "font-name" font-size :font-style)
;; e.g., ("helvetica" 16 :plain)
;;
(defun STRING-WIDTH (String &optional Font-Spec)
"Returns the width in pixels of String, as if it were displayed in the font,
size, and style of font-spec."
(if (null font-spec)
(format t "No spec!")
;; NSDictionary* attributes = [NSDictionary dictionaryWithObjectsAndKeys: font, nil];
;; NSString foo = String;
;; Size = foo :sizeWithAttributes attributes
(let ((size (float (second font-spec))))
(with-cstrs ((c-font-name (first font-spec))
(c-string String))
(with-autorelease-pool
(let* ((tempname (ccl::send (@class ns-string) :string-with-c-string c-font-name))
(fontname (ccl::send tempname 'capitalized-string))
(nsstring (ccl::send (@class ns-string) :string-with-c-string c-string))
(font (ccl::send (ccl::send (find-class 'ns:ns-font) :font-with-name fontname :size size) 'retain)))
(rlet ((glyph-size :ize))
(let ((dict (ccl::send (ccl::send (find-class 'ns:ns-dictionary) :dictionary-with-objects-and-keys font (:id #&NSFontAttributeName :address (%null-ptr))) 'retain)))
(ccl::send/stret glyph-size nsstring :size-with-attributes dict)
(let ((width (ccl::pref glyph-size :ize.width)))
(ccl::send dict 'release)
(ccl::send font 'release)
width)))))))))
But the results are very cool!
Comments